Updates
August 12, 2020: Voiceflow implemented ability to use external libraries in the code block. “var” was removed from the code block example, as the variable is already initialized.
May 30, 2019: This tutorial is for generating random numbers that can repeat. In this case each new number generated is independent of the previous number’s generation. An example is rolling dice.
A second tutorial has been published for generating non-repeating random numbers. In this case all the numbers in the range will be used before there are repeats. Review tutorial “Voiceflow Non-Repeating Random Number Generator” for this type of functionality.
Level: Intermediate
This tutorial assumes you are familiar with the basics of creating Alexa skills using Voiceflow, including the use of variables, the SET block and the SPEAK block. This tutorial introduces the new CODE block, and illustrates how to add some Javascript which will generate a random number.
If you haven’t developed an Amazon Alexa skill before, or haven’t used Voiceflow, the following are recommended as prerequisites:
- Set up an Amazon Developer account. It is free:
Amazon Alexa Developer Home Page
2. Set up a Voiceflow Account. They offer a generous free tier from which you can design, develop and publish Amazon Alexa skills:
3. Try some of the Voiceflow University tutorials and practice making a skill or two. There is an introductory video series, along with extensive reference documentation.
Voiceflow Basics Tutorial Series
4. Join the Voiceflow User’s Group on Facebook. The community is very supportive should you have questions or issues when building skills, as well as a lot of tips, techniques and camaraderie.
Introduction
This tutorial shows how to add Javascript code to a Voiceflow CODE block which will generate random number integers between ONE and a specified maximum value.
There are a myriad of uses for random numbers in voice skills. Uses can range from mimicking dice rolls in a game to passing them into API calls to retrieve a set of values from a row in a database.
For this tutorial, the code inside the block has two variables, one for the maximum number (maxNo), and the other for the generated random number (randomNo).
However the upper limit value can be hard coded as well, depending on your needs. For example, a value of “6” can be used directly in the formula in order to generate a random integer between one and six, if all you need to do is mimic a six-sided dice roll for your skill.
Limitation – Formula supports randomly generated integers ONE through a specified maximum number only
The Javascript formula used for this tutorial will generate a random integer between ONE and a specified maximum value (inclusive). If a random value between ZERO and a maximum value is needed, or if alternate minimum values need to be specified, different formulas will be needed.
A variety of formulas can be found by searching Javascript-oriented websites, and you can also ask your peers in the Voiceflow Facebook Group for assistance.
Javascript Reference Documentation
Below is a link to the reference documentation for the javascript code used for this tutorial. Review the “More Examples” section for code samples:
w3schools.com JavaScript random() Method
Demonstration Skill
The demo skill shows how to configure and test the code. Here is our demo skill layout on the Voiceflow project canvas:
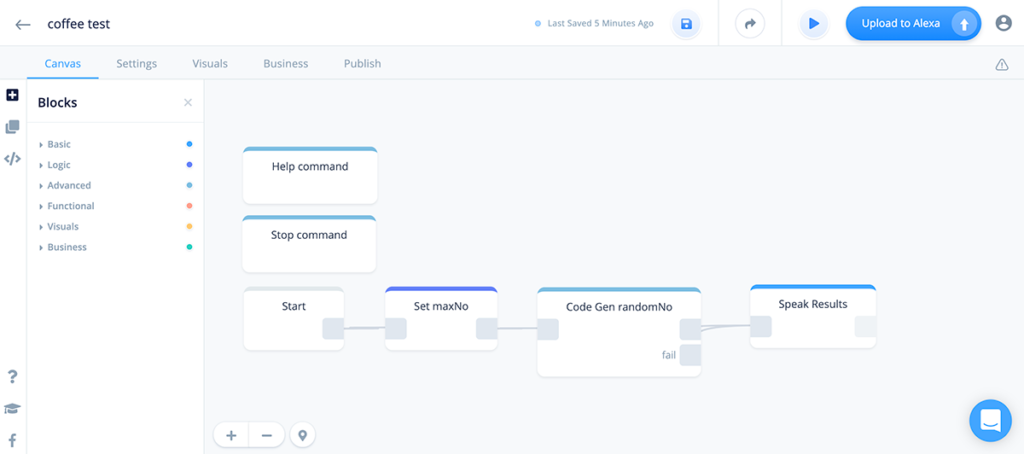
During this tutorial, we will configure each block, from left to right, as well as upload to Alexa for testing. Specifically, we will be working with the SET block, the CODE block, and the SPEAK block. If you wish, you can either drag all of the blocks onto the canvas right away, or add them one at a time and connect them as we proceed through the tutorial.
PRO TIP: During implementation for a skill going into production, an excellent option is to implement the CODE block in a sub flow. The sub flow containing the CODE block can more easily be called multiple times throughout a larger skill using FLOW blocks.
Variable Configuration
For this tutorial, the following variables are configured in Voiceflow. Variables maxNo and randomNo are created. maxNo will store the upper-end of the random number range. randomNo will store the generated random number. These can be global or local depending on your needs:
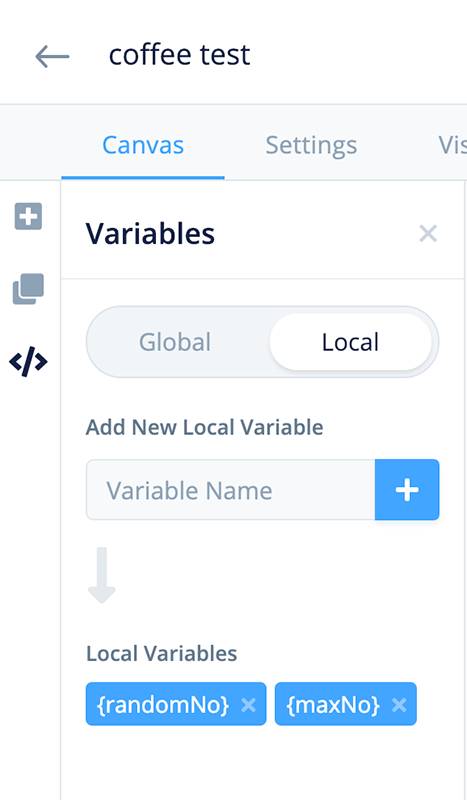
SET Block Configuration
For this tutorial, a SET block is used to assign a value to the maxNo variable. Of course, the variable can be set in a variety of ways, and with a variety of values in a skill destined for publication.
The maxNo variable is used to identify the upper limit for the random number (i.e. a value between 1 and maxNo), and should be an integer. For this demo, 75 is used.
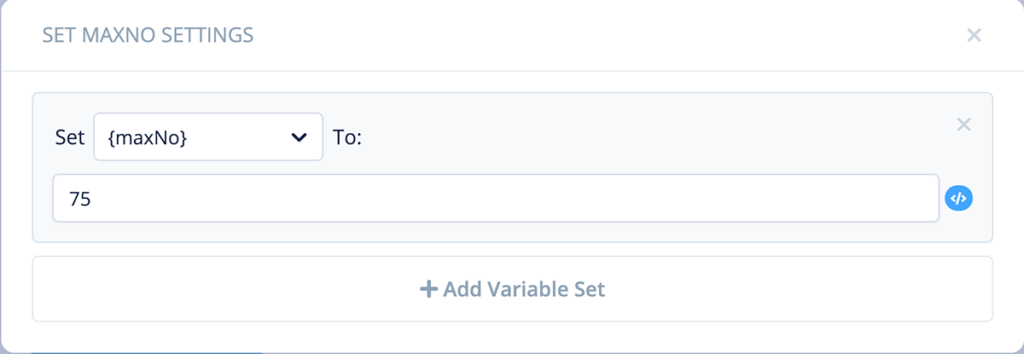
Introducing the new CODE Block
At the time of this posting, Voiceflow just introduced the new CODE Block. Javascript can be implemented inside this block. It can be found in the Blocks/Advanced section in the left-hand panel, as shown below:
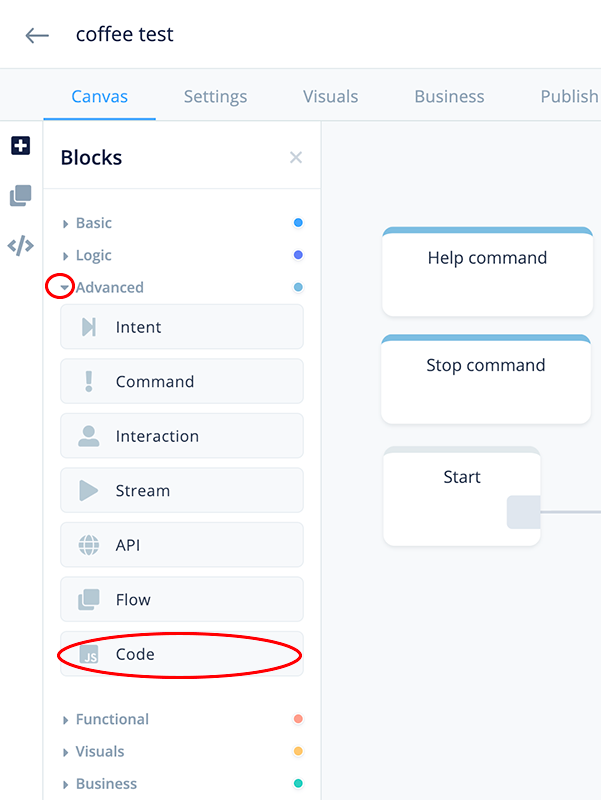
CODE Block Configuration
Here is our Code block configuration with our Javascript random number formula:

Here is the text of the comment (in line 1) and the code (in line 2), of you wish to copy / paste:
//Random number generator for values 1 through maxNo
randomNo = Math.floor((Math.random() * maxNo) + 1);
SPEAK Block Configuration
A SPEAK block is used for testing, to help confirm the two variables have expected results:
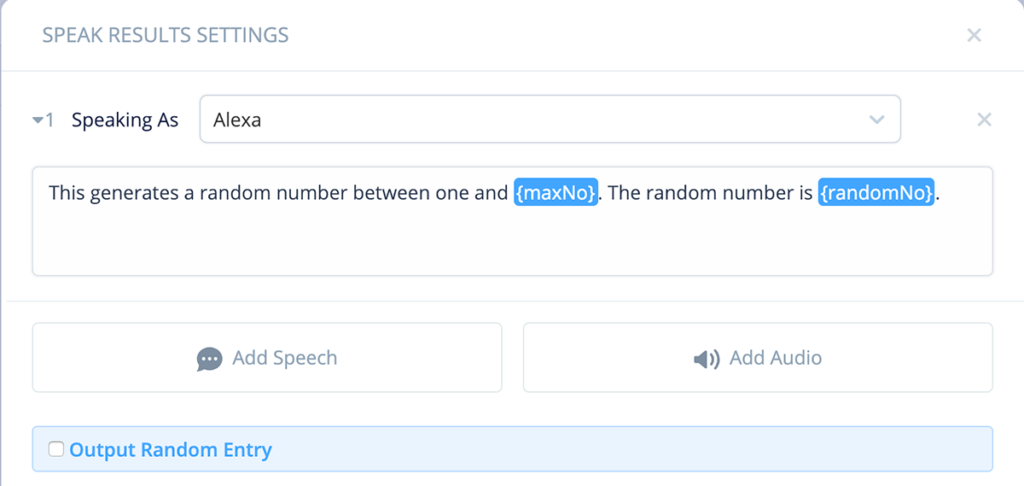
Voiceflow Tests
The following are three tests using the Voiceflow testing tool, with Debug Mode on.
Test 1:
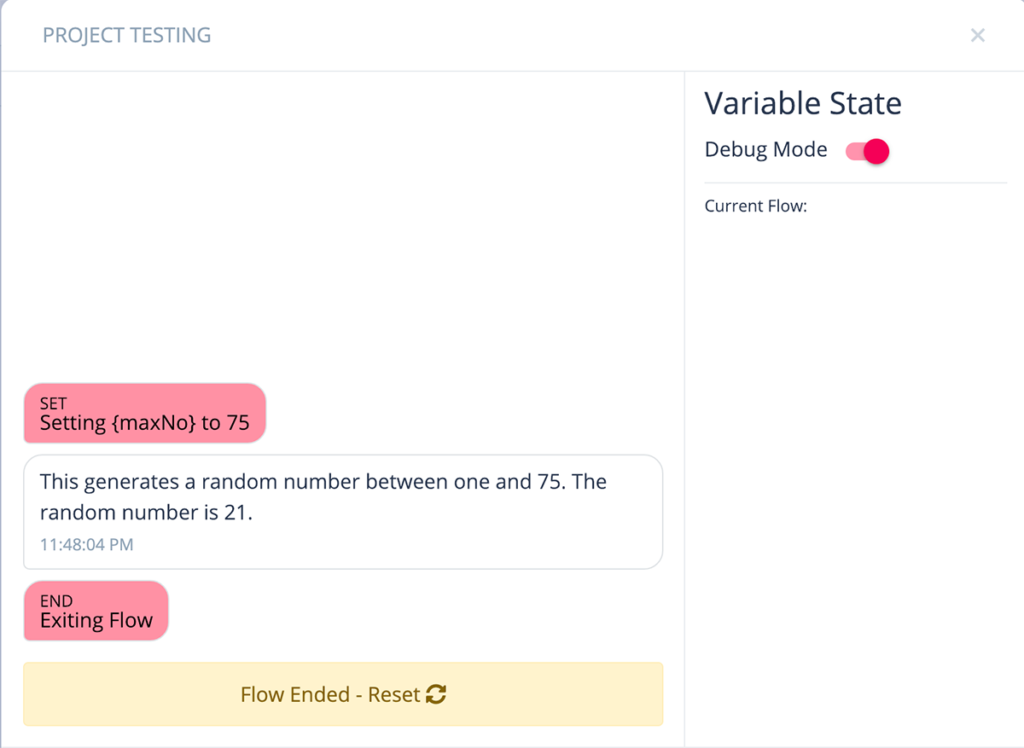
Test 2:
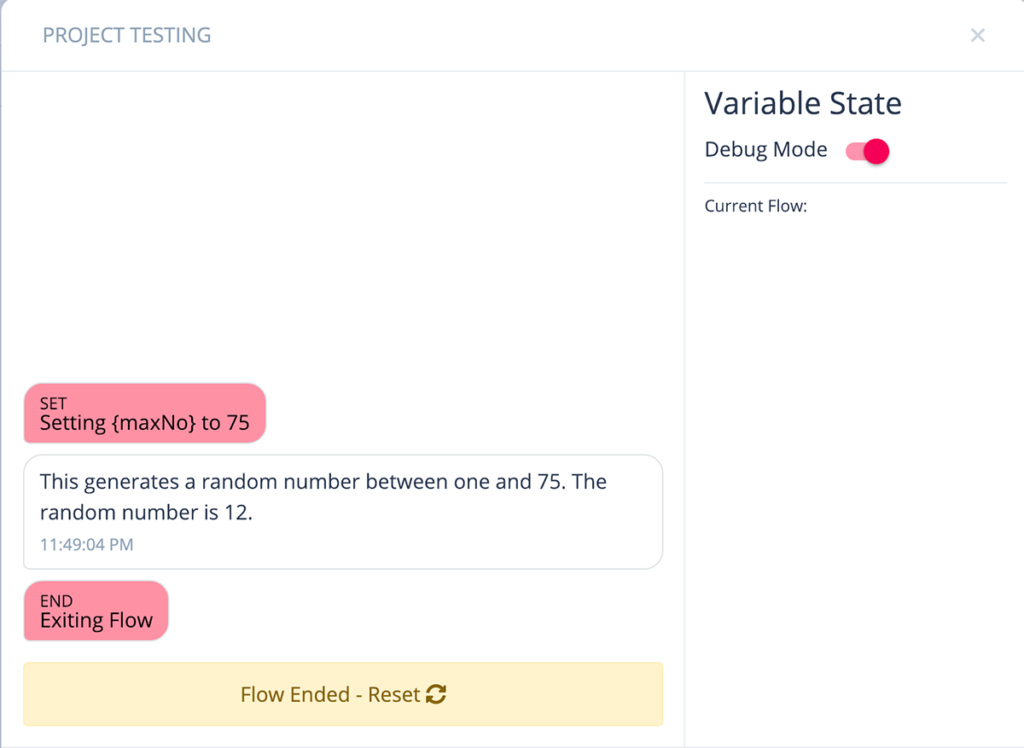
Test 3:
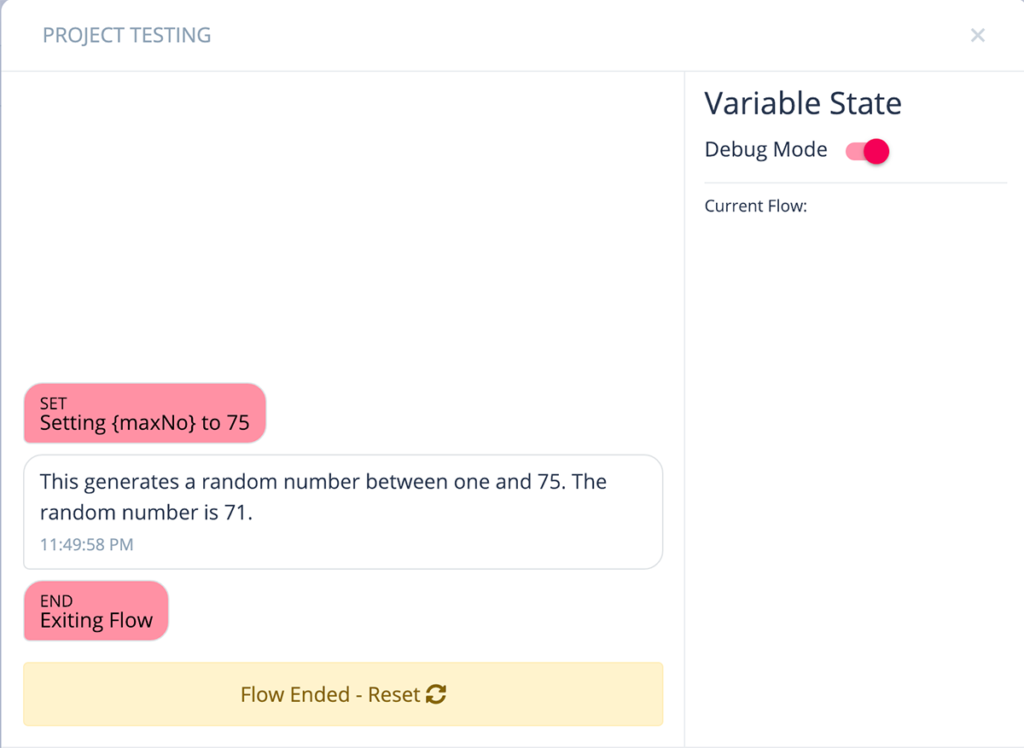
The above three tests resulted in generating numbers between 1 and 75, and as such are successful.
Alexa Developer Console Tests
The next three test are performed in the Alexa Developer Console.
Test 1:
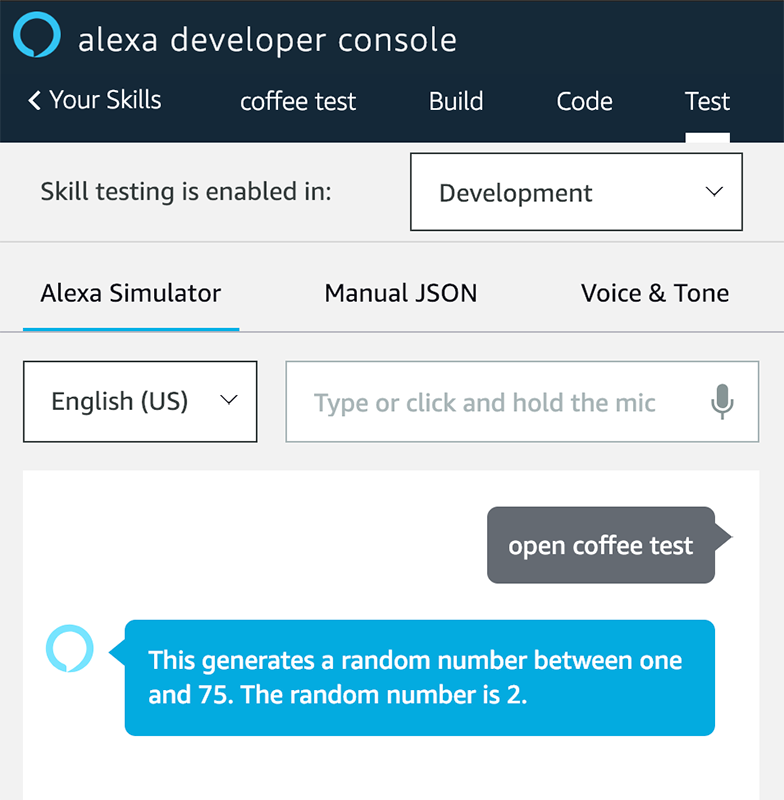
Test 2:
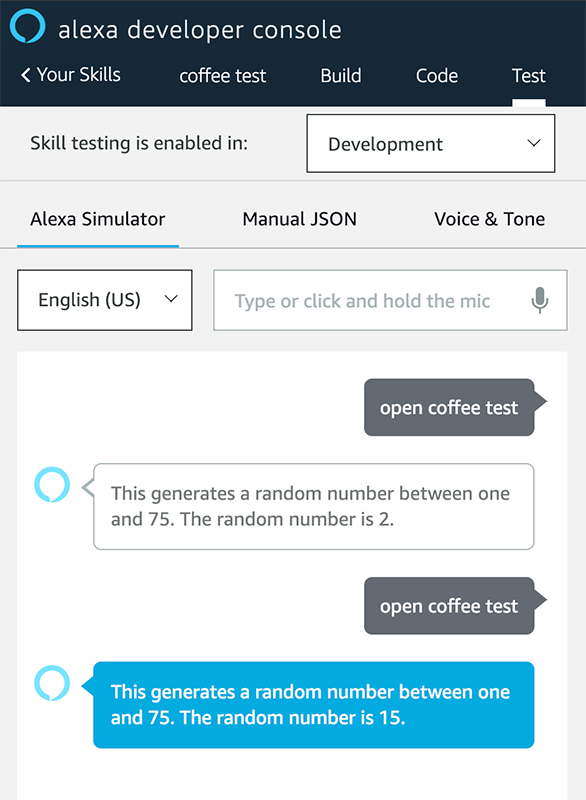
Test 3:
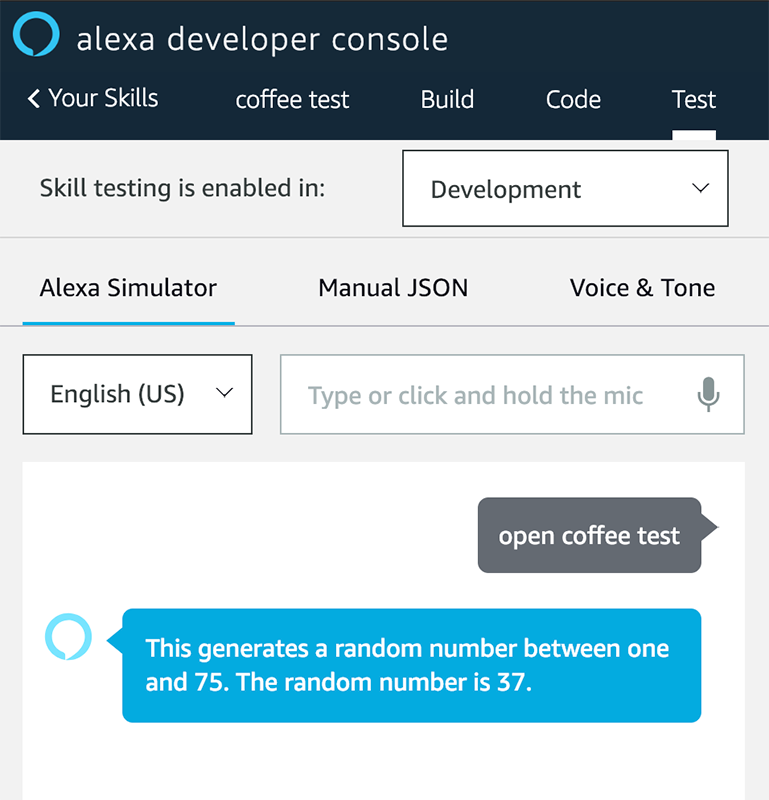
During testing via the Alexa Developer Console, all three results were within the range of 1 and 75, and are considered successful.
Conclusion
The above is a simple example of a way to use the Voiceflow CODE Block. This block, combined with the power of Javascript, can provide more opportunities when designing and developing voice skills.
Thank you for reading, and happy skill building!
Credits
Header photo by Alex Chambers on Unsplash.